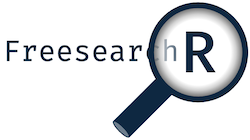
Alternative pivoting method for easily pivoting based on name pattern
Source:R/wide2long.R
wide2long.Rd
This function requires and assumes a systematic naming of variables. For now only supports one level pivoting. Adding more levels would require an added "ignore" string pattern or similarly. Example 2.
Usage
wide2long(
data,
pattern,
type = c("prefix", "infix", "suffix"),
id.col = 1,
instance.name = "instance"
)
Examples
data.frame(
1:20, sample(70:80, 20, TRUE),
sample(70:100, 20, TRUE),
sample(70:100, 20, TRUE),
sample(170:200, 20, TRUE)
) |>
setNames(c("id", "age", "weight_0", "weight_1", "height_1")) |>
wide2long(pattern = c("_0", "_1"), type = "suffix")
#> id age instance weight height
#> 1 1 71 0 95 NA
#> 2 1 NA 1 98 179
#> 3 2 72 0 77 NA
#> 4 2 NA 1 98 182
#> 5 3 70 0 85 NA
#> 6 3 NA 1 74 197
#> 7 4 71 0 83 NA
#> 8 4 NA 1 82 199
#> 9 5 72 0 90 NA
#> 10 5 NA 1 88 179
#> 11 6 75 0 94 NA
#> 12 6 NA 1 94 175
#> 13 7 74 0 75 NA
#> 14 7 NA 1 100 179
#> 15 8 71 0 91 NA
#> 16 8 NA 1 83 195
#> 17 9 78 0 77 NA
#> 18 9 NA 1 96 176
#> 19 10 79 0 90 NA
#> 20 10 NA 1 92 187
#> 21 11 78 0 93 NA
#> 22 11 NA 1 96 188
#> 23 12 71 0 72 NA
#> 24 12 NA 1 73 193
#> 25 13 71 0 77 NA
#> 26 13 NA 1 89 187
#> 27 14 76 0 74 NA
#> 28 14 NA 1 100 192
#> 29 15 73 0 83 NA
#> 30 15 NA 1 96 190
#> 31 16 75 0 96 NA
#> 32 16 NA 1 82 183
#> 33 17 71 0 82 NA
#> 34 17 NA 1 72 192
#> 35 18 76 0 88 NA
#> 36 18 NA 1 77 195
#> 37 19 78 0 72 NA
#> 38 19 NA 1 93 184
#> 39 20 75 0 97 NA
#> 40 20 NA 1 98 198
data.frame(
1:20, sample(70:80, 20, TRUE),
sample(70:100, 20, TRUE),
sample(70:100, 20, TRUE),
sample(170:200, 20, TRUE)
) |>
setNames(c("id", "age", "weight_0", "weight_a_1", "height_b_1")) |>
wide2long(pattern = c("_0", "_1"), type = "suffix")
#> id age instance weight weight_a height_b
#> 1 1 79 0 75 NA NA
#> 2 1 NA 1 NA 71 171
#> 3 2 74 0 93 NA NA
#> 4 2 NA 1 NA 97 199
#> 5 3 75 0 95 NA NA
#> 6 3 NA 1 NA 81 192
#> 7 4 70 0 97 NA NA
#> 8 4 NA 1 NA 75 195
#> 9 5 70 0 77 NA NA
#> 10 5 NA 1 NA 83 191
#> 11 6 75 0 90 NA NA
#> 12 6 NA 1 NA 95 178
#> 13 7 75 0 76 NA NA
#> 14 7 NA 1 NA 78 200
#> 15 8 79 0 72 NA NA
#> 16 8 NA 1 NA 76 185
#> 17 9 75 0 79 NA NA
#> 18 9 NA 1 NA 88 195
#> 19 10 78 0 70 NA NA
#> 20 10 NA 1 NA 74 199
#> 21 11 70 0 99 NA NA
#> 22 11 NA 1 NA 86 176
#> 23 12 77 0 74 NA NA
#> 24 12 NA 1 NA 78 174
#> 25 13 75 0 74 NA NA
#> 26 13 NA 1 NA 91 192
#> 27 14 70 0 73 NA NA
#> 28 14 NA 1 NA 81 176
#> 29 15 79 0 80 NA NA
#> 30 15 NA 1 NA 91 176
#> 31 16 72 0 78 NA NA
#> 32 16 NA 1 NA 94 181
#> 33 17 77 0 71 NA NA
#> 34 17 NA 1 NA 82 181
#> 35 18 80 0 97 NA NA
#> 36 18 NA 1 NA 81 200
#> 37 19 80 0 79 NA NA
#> 38 19 NA 1 NA 72 195
#> 39 20 74 0 71 NA NA
#> 40 20 NA 1 NA 77 171
# Optional filling of missing values by last observation carried forward
# Needed for mmrm analyses
long_missings |>
# Fills record ID assuming none are missing
tidyr::fill(record_id) |>
# Grouping by ID for the last step
dplyr::group_by(record_id) |>
# Filling missing data by ID
tidyr::fill(names(long_missings)[!names(long_missings) %in% new_names]) |>
# Remove grouping
dplyr::ungroup()
#> Error: object 'long_missings' not found